Javascript Infused Bookmarks
Thu, Mar 20, 2025
I have often thought about writing my own browser plugin to manipulate CSS/DOM or automate tasks.
Today, I had to delete local storage very frequently while debugging a web application.
I thought it simply couldn’t be that I needed more than one click to delete a website’s data in the browser, not to mention installing a plugin for it.
I quickly asked GPT how to delete website data in the browser more efficiently. The result was predictably disappointing: use dev tools or browser settings. Somehow, my bookmark bar was smiling at me at that moment, so I asked GPT if it was possible to delete all data of the current tab with a bookmark.
Et voilà, bookmarks can execute JavaScript—why didn’t I think of that earlier? Just add a new bookmark and inject your JavaScript code as a URL. A JavaScript-infused bookmark is called a bookmarklet (ill-named, in my opinion).
The format is always:
javascript:(function(){
// statements
})();
Before saving your code to the bookmark, make sure to minify the script and remove any comments.
My use cases so far
Autofill credentials
javascript:(function () {
if (window.location.host === "localhost:7857") {
let u = document.querySelector('input[type="text"]');
let p = document.querySelector('input[type="password"]');
if (u) {
u.value = "admin";
}
if (p) {
p.value = "password";
}
console.log("Login fields auto-filled for localhost:7857.");
} if (window.location.host === "localhost:1337") {
let u = document.querySelector('input[type="text"]');
let p = document.querySelector('input[type="password"]');
if (u) {
u.value = "superadmin";
}
if (p) {
p.value = "password";
}
console.log("Login fields auto-filled for localhost:1337");
}
})();
Bypass paywalls with archive.is
javascript:(function () {
window.location='https://archive.is/'+encodeURIComponent(window.location.href);
})();
Delete local storage data of the current tab
This works for my needs so far.
Be aware that cookie handling could be improved.
javascript:(function () {
let origin = window.location.origin;
if (!origin) {
alert('No site detected!');
return;
}
(async function () {
if (window.caches) {
await caches.keys().then(keys => keys.forEach(key => caches.delete(key)));
} else {
console.warn('Cache API is not available.');
}
localStorage.clear();
sessionStorage.clear();
if (window.indexedDB && indexedDB.databases) {
indexedDB.databases().then(dbs => dbs.forEach(db => indexedDB.deleteDatabase(db.name)));
} else {
console.warn('IndexedDB API is not available.');
}
document.cookie.split(';').forEach(cookie => {
document.cookie = cookie.replace(/^ +/, '').replace(/=.*/, '=;expires=' + new Date(0).toUTCString() + ';path=/;domain=' + location.hostname);
});
}());
})();
Bypass annoying website overlays
Since I refuse to use tracking banners that don’t provide a direct opt-out option, bookmarklets are a perfect way to make these websites more user-friendly.
javascript:(function () {
let hostname = window.location.hostname;
// Restore scrolling
document.body.style.setProperty('position', 'unset', 'important');
document.body.style.setProperty('overflow', 'unset', 'important');
// Remove fixed elements (direct children of body)
document.querySelectorAll('body > *').forEach(el => {
if (getComputedStyle(el).position === 'fixed') {
el.style.setProperty('display', 'none', 'important');
}
});
// Check for morgenpost.de and hide ad tracking overlay
if (hostname.includes("morgenpost.de")) {
console.log("Applying specific rules for morgenpost.de");
let bodyChildren = document.body.children;
if (bodyChildren.length > 0) {
bodyChildren[0].style.setProperty('display', 'none', 'important');
}
if (bodyChildren.length > 1) {
bodyChildren[1].style.setProperty('display', 'none', 'important');
}
}
if (hostname.includes("duden.de")) {
console.log("Applying specific rules for duden.de");
// Select the div with an ID that starts with 'sp_message_container_'
const messageContainer = document.querySelector('div[id^="sp_message_container_"]');
// If the element exists, remove it
if (messageContainer) {
messageContainer.remove();
}
}
})();
Without comments.
javascript:(function () {
let hostname = window.location.hostname;
document.body.style.setProperty('position', 'unset', 'important');
document.body.style.setProperty('overflow', 'unset', 'important');
document.querySelectorAll('body > *').forEach(el => {
if (getComputedStyle(el).position === 'fixed') {
el.style.setProperty('display', 'none', 'important');
}
});
if (hostname.includes("morgenpost.de")) {
console.log("Applying specific rules for morgenpost.de");
let bodyChildren = document.body.children;
if (bodyChildren.length > 0) {
bodyChildren[0].style.setProperty('display', 'none', 'important');
}
if (bodyChildren.length > 1) {
bodyChildren[1].style.setProperty('display', 'none', 'important');
}
}
if (hostname.includes("duden.de")) {
console.log("Applying specific rules for duden.de");
const messageContainer = document.querySelector('div[id^="sp_message_container_"]');
if (messageContainer) {
messageContainer.remove();
}
}
})();
Website defacing locally in browser
If you want to quickly change the text of a website for screenshots etc., this can come in very handy. Changes will vanish on reload.
javascript:(function() {
document.designMode = "on";
})();
Bookmarklets in action
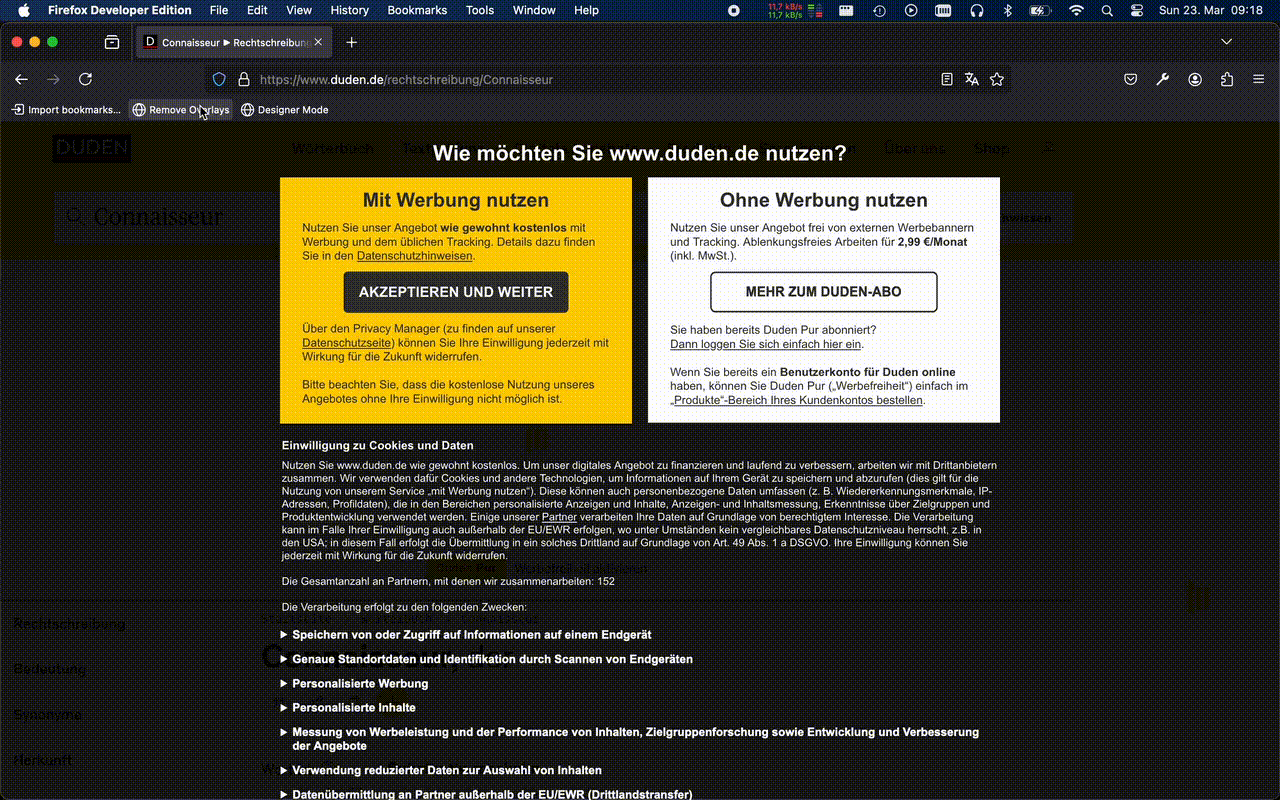